From Rails app to to iOS app with Hotwire Native #1
Documenting my experience taking a new Rails app (Missionbase) to the iOS app store.
Days 1-3
Saturday, May 17, 2025 to Monday, May 19, 2025
Progress
- Set up Xcode project for Missionbase
- Set up the project with Hotwire Native for iOS dependencies
- Got app running in simulator and on my iPhone.
- Got app running using both production and local development servers.
- Tried to customize the top UI navigation title. It's not working the way I wanted it so I've left it to the default for now.
- Implemented navigation tabs. This was the most straightforward part of anything so far.
- Sort of got path-configurations working.
Thoughts
- AI isn't very helpful for this. I would think it's likely because Hotwire is still quite new and there isn't a whole lot of documentation around it. But unfortunately it hasn't been able to help me accomplish anything.
- A lot of unclarity for any customization. The basic Hotwire Native iOS walkthrough helped get me set up and get the app running in development mode on my iPhone, but making even minor customizations hasn't been easy. From what I'm finding so far, it seems like knowing Swift really is a requirement if you want to do anything beyond the defaults.
- Xcode is awful. I've found using Xcode as an editor to be downright awful so I took some time today to set up Neovim for my Xcode projects. I found a good plugin called xcodebuild.nvim which allows me to perform builds, run and clear using commands right within Neovim. This has already immensely improved my development happiness.
- I'm going to have to learn Swift. Coming into this I had the idea that I might be able to get by without doing much iOS development. Wrong. While getting a working app was pretty easy, getting this app approved in the app store seems very unlikely without knowing a good amount of Swift. This is largely because this app is a subscription-based app, so I have to figure out Apple's subscription payments side of it. Perhaps if I was working with just a free app, this would be much easier.
Issues I've run into
- Dev server issue. Hooking into the Rails local development server didn't work at first. Had to add a specific entry to
Info.plist
in order to be able to have the app hook up to the local development server without SSL. And then just usinglocalhost:3000
when using the app on my iPhone because of course the iPhone has to have the IP address of the Mac where the Rails dev server is. So I set up an entry inInfo.plist
to allow non-SSL for the app to the dev server. This means that the remote URL for the app server is192.168.1.56:3000
. You'll also need to bind the IP address usingbin/rails server -b 0.0.0.0 -p 3000
so that the app is accessible from within your network by other devices. - Can't customize the top navigation bar. Hotwire, by default, sets up the iOS UI navigation title to use whatever the
<title>
tag has set for the page in the Rails app. This is simple, but if you take a look at the screenshot, it means there's redundant titles. There's an easy to to handle this even just using Tailwind, but the issue I have is that there are other views in the app where I would like to have more information in that title area. For a Task for example, I would like to have the title of the task, along with the project it belongs to as a subheading. But I haven't been able to attain this so far. It seems like I'll need to set up some sort custom navigation views (or something).
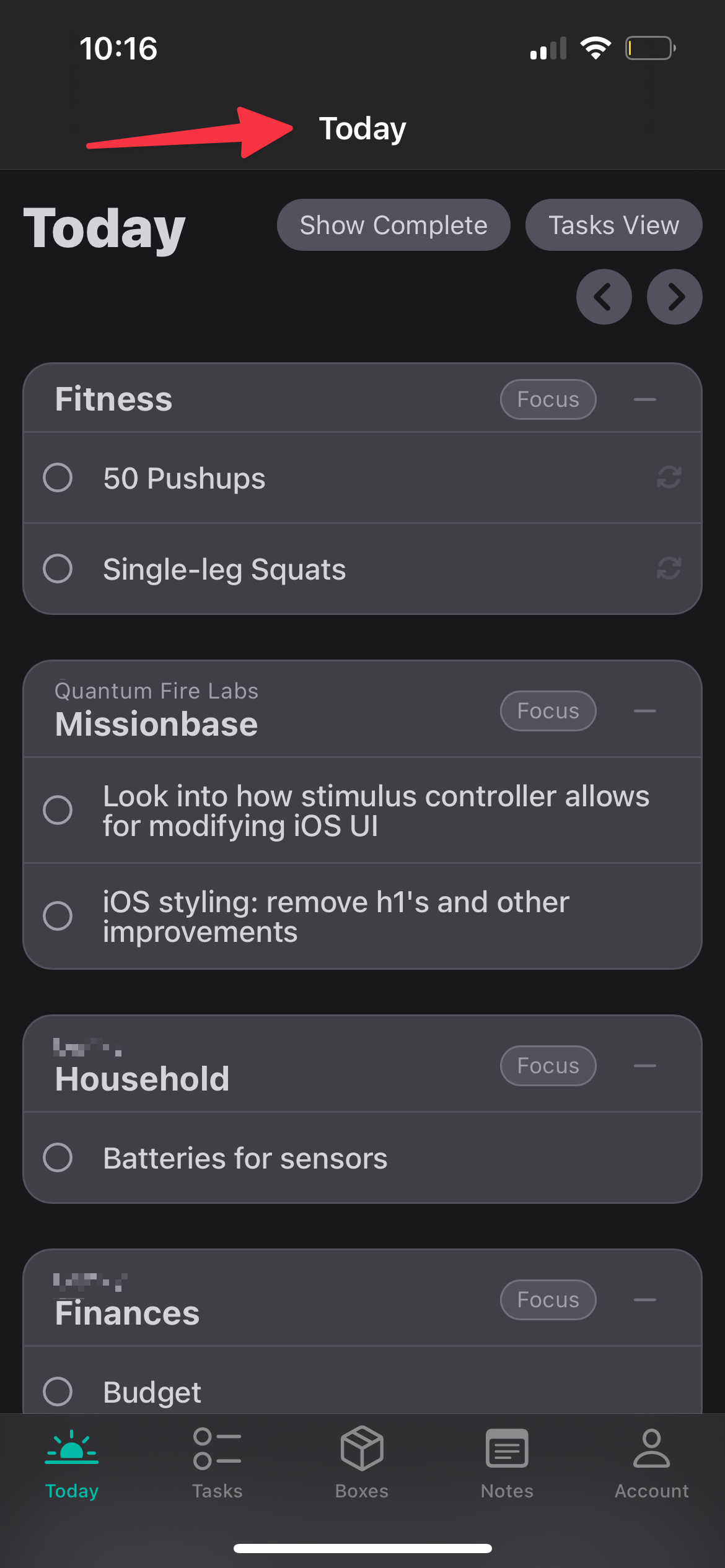
- Handling subscriptions is very unclear. I began looking into how to handle subscriptions in the app and again... it looks like I'm going to really have to dive into Swift development. Not only will I need to be able to set up the ability for a user to subscribe, but I'll also need to connect the Rails server so that subscription details stay in sync with whatever is happening on the Apple subscription-side of things when a user subscribes. Or... I wait it it and see if Apple drops the in-app purchase requirement for subscription based apps in Canada like they have in the US.
- Still don't quite understand what's going with path configurations. I mean, in theory I understand the concept, but in practicality of what's actually happening in the app, it seems to be behaving differently than what I'm intending. There are a couple views that don't seem to "stack" or use the modal the way I'm instructing them to.